Achieve Python Asyncio Mastery:
Your Ultimate Guide To
The High-Level Asyncio API
Embrace modern asynchronous programming for
scalable Python application development.
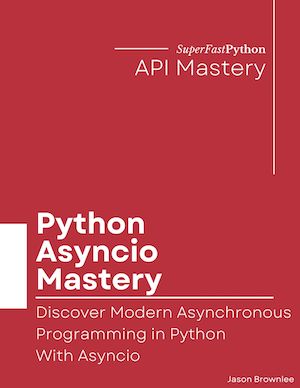
Get on top of the latest asyncio features
like TaskGroup, Barrier, and timeout()
Asynchronous programming is built into Python.
The language directly supports coroutines as first-class objects with the โasyncโ and โawaitโ expressions for asynchronous programming. The asyncio module provides tools for creating and managing asynchronous tasks and for developing non-blocking I/O client and server programs.
Asyncio is not coming, itโs here.
Skills in asyncio are in demand and the demand is growing. Why?
Asynchronous programming and asyncio are how we develop modern scalable applications in Python. This paradigm dominates modern web development, API development, and network programming, and few Python programs do not touch on these areas.
Developing concurrent programs using coroutines and the asyncio module API can be very challenging, especially for Python developers who are new to asynchronous programming.
Introducing: โPython Asyncio Masteryโ. A new book designed to teach you asyncio in Python, super fast!
You will get in-depth tutorials showing you how to develop asyncio programs on advanced topics, including:
- Tasks: How to define, schedule, execute, check the status, and get results from asynchronous tasks.
- Multiple Tasks: How to manage groups of asynchronous tasks, including waiting for tasks, getting results, grouping tasks, and using timeouts.
- Advanced Tasks: How to use more advanced features of tasks such as shielding, sleeping, waiting for, and executing blocking tasks.
- Structures: How to define, create, and use asynchronous iterators, generators, context managers, and queues.
- Synchronization: How to safely synchronize and coordinate the behavior of coroutines with mutex locks, semaphores, barriers, and more.
- Subprocesses: How to run commands and perform non-blocking inter-process communication with subprocesses.
- Streams: How to develop clients and servers with socket programming and perform non-blocking reads and writes.
Each tutorial is carefully designed to teach you one critical aspect of how to use asyncio in your Python programs.
Table of Contents
The book is divided into 7 parts and 36 chapters, they are:
Part I: Asyncio
- Chapter 01: What is Asyncio
- Chapter 02: What is a Coroutine
- Chapter 03: Asyncio Hello World
Part II: Asyncio Tasks
- Chapter 04: What Are Tasks
- Chapter 05: Task Name
- Chapter 06: Task Status
- Chapter 07: Task Cancellation
- Chapter 08: Task Results
- Chapter 09: Task Exceptions
- Chapter 10: Task Done Callbacks
- Chapter 11: Task Done Callbacks
- Chapter 12: Main and Current Task
- Chapter 13: All Tasks
Part III: Multiple Tasks
- Chapter 14: Gather Tasks
- Chapter 15: Wait on Tasks
- Chapter 16: Tasks As Completed
- Chapter 17: Task Group
- Chapter 18: Tasks with Timeouts
Part IV: More Tasks
- Chapter 19: Shield Tasks
- Chapter 20: Sleep Tasks
- Chapter 21: Wait For Tasks
- Chapter 22: Blocking Tasks
Part V: Structure
- Chapter 23: Asynchronous Iterators
- Chapter 24: Asynchronous Generators
- Chapter 25: Asynchronous Context Managers
- Chapter 26: Asynchronous Queues
Part VI: Synchronization
- Chapter 27: Locks
- Chapter 28: Events
- Chapter 29: Conditions
- Chapter 30: Semaphores
- Chapter 31: Barriers
Part VII: Streams
- Chapter 32: Subprocesses
- Chapter 33: Streams
- Chapter 34: Check Website Status
- Chapter 35: Servers
- Chapter 36: Chat Client and Server
Sample Pages
Below are some sample pages from the book, click to enlarge.
BONUS: Asyncio Script Library
โฆyou also get 103 fully working asyncio scripts
Each example presented in the book is standalone meaning that you can copy and paste it into your project and use it immediately.
You get one Python script (.py) for each example provided in the book.
Your Asyncio Script Library is organized by chapter coving topics such as:
Asyncio Task
- Examples of creating, naming and querying the status asyncio tasks
- Examples of canceling tasks
- Examples of getting results and exceptions from tasks
Multiple Tasks
- Examples of executing tasks concurrently with gather(), wait() and as_completed()
- Examples of managing tasks with a TaskGroup
- Examples of managing timeouts for blocks with timeout()
Advanced Tasks
- Examples of shielding and sleeping tasks
- Examples of waiting for tasks with a timeout
- Examples of running blocking tasks from asyncio
Structures
- Examples of async iterators, generators, and context managers
- Examples us async queues and producer-consumer tasks
Synchronization
- Examples of using mutex locks, events, and condition variables.
- Examples of using semaphores and barriers
Streams
- Examples of non-blocking I/O with asyncio streams
- Case Studies using client and server socket programming.
This means that you can follow along and compare your answers to a known working implementation of each example in the provided asyncio library.
This helps a lot to speed up your progress when working through the details of a specific asyncio task.
Your Learning Outcomes
You will transform you into a Python developer who can confidently build applications with asynchronous programming and the high-level asyncio API.
1. You will know how to confidently develop Python programs with the asynchronous programming paradigm, including:
- How to structure and run an asyncio programs.
- How to create and execute coroutines.
- How to use the expressions added to the Python language to implement cooperative multitasking.
2. You will know how to confidently create, run, and query asyncio tasks, including:
- How to create and schedule coroutines as tasks and run them in the foreground or background.
- How to query task status and assign them meaningful names.
- How to cancel tasks, handle task cancellation, and check if a task has been canceled.
- How to retrieve results from tasks and exceptions raised in tasks.
- How to add and mange done callback functions executed by tasks.
- How to introspect the current and all running tasks and know the role and importance of the main task.
3. You will know how to confidently manage groups of asyncio tasks, including:
- How to execute multiple tasks concurrently and retrieve their results once they are all done.
- How to wait for a condition on a group of tasks, such as all done, first done, or first to fail.
- How to process task results in the order that tasks are completed rather than the order they were issued.
- How to define a related collection of tasks as a group and operate upon the group, such as cancel all if one fails.
- How to execute tasks and blocks of asyncio code with timeouts.
4. You will know how to confidently implement more complex task management, including:
- How to shield tasks from cancellation.
- How to yield control with sleep to allow scheduled tasks to execute.
- How to wait for a single task to complete with a timeout.
- How to execute a blocking task without stopping the asyncio event loop.
5. You will know how to confidently use asynchronous control flow and data structures, including:
- How to develop and traverse asynchronous iterators and generators
- How to develop and use asynchronous context managers.
- How to communicate between coroutines and tasks using queues.
6. You will know how to confidently synchronize the behavior between tasks, including:
- How to use mutex locks to avoid race conditions and deadlocks, ensuring coroutine safety.
- How to synchronize behavior with shared events and use the wait/notify pattern with condition variables.
- How to limit access to shared resources by tasks using semaphores.
- How to coordinate behavior between tasks using barriers.
7. You will know how to confidently communicate with other processes and networked programs efficiently, including:
- How to create, manage, and perform non-blocking reads and writes with subprocesses.
- How to read and write with non-blocking network sockets.
- How to develop an asynchronous website status-checking application.
- How to develop and manage asynchronous socket servers
- How to develop an asynchronous group chat application.
Introducing:
โPython Asyncio Masteryโ
Discover Modern Asynchronous Programming In Python With Asyncio
โPython Asyncio Masteryโ is my new book that will teach you how to use the high-level async API in the standard library, from scratch.
This book distills what you need to know for application development with the asyncio API, super fast.
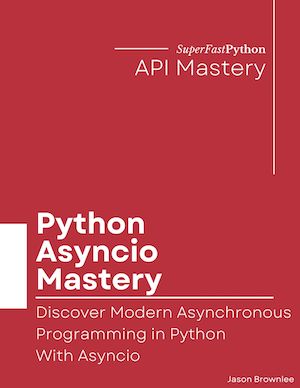
Technical Details:
- 36 tutorialsย taught by example with full working code listings.
- 103 .py code filesย included that you can use as templates
- 388 pagesย for on-screen reading open next to your editor.
- 2 formats (PDF and EPUB)ย for screen, tablet, and kindle reading.
- 1.5 megabyteย .zip download that contains the ebook and all source code.
โPython Asyncio Masteryโ will lead you on the path from a Python developer interested in asyncio to a developer who can confidently develop asyncio applications.
Itโs exactly how I would teach you the asyncio API if we were sitting together, pair programming.
Choose Your Package:
BOOK
You get the book:
- Python Asyncio Mastery
PDF and EPUB formats
Includes all source code files
BOOKSHELF
BEST VALUE
You get everything (15+ books):
- Threading Jump-Start
- Multiprocessing Jump-Start
- Asyncio Jump-Start
- ThreadPool Jump-Start
- Pool Jump-Start
- ThreadPoolExecutor Jump-Start
- ProcessPoolExecutor Jump-Start
- Threading Interview Questions
- Multiprocessing Interview Questions
- Asyncio Interview Questions
- Executors Interview Questions
- Concurrent File I/O
- Concurrent NumPy
- Python Benchmarking
- Python Asyncio Mastery
Bonus, you also get:
- Concurrent For Loops Guide
- API Mind Maps (4)
- API Cheat Sheets (7)
That is $210 of Value!
(you get a 10.95% discount)
All prices are in USD.
(also get the book from Amazon, GumRoad, and GooglePlay stores)
About the Author
Hi, Iโm Jason Brownlee, Ph.D.
Iโm a Python developer, husband, and father to two boys.
I want to share something with you.
I am obsessed with Python concurrency, but I wasnโt always this way.
My background is in Artificial Intelligence and I have a few fancy degrees and past job titles to prove it.
You can see my LinkedIn profile here:
- Jason Brownlee LinkedIn Profile
(follow me if you like)
So what?
Well, AI and machine learning has been hot for the last decade. I have spent that time as a Python machine learning developer:
- Working on a range of predictive modeling projects.
- Writing more than 1,000+ tutorials.
- Authoring over 20+ books.
Thereโs one thing about machine learning in Python, your code must be fast.
Really fast.
Modeling code is already generally fast, built on top of C and Fortran code libraries.
But you know how it is on real projectsโฆ
You always have to glue bits together, wrap the fast code and run it many times, and so on.
Making code run fast requires Python concurrency and I have spent most of the last decade using all the different types of Python concurrency available.
Including threading, multiprocessing, asyncio, and the suite of popular libraries.
I know my way around Python concurrency and I am deeply frustrated at the bad wrap it has.
This is why I started SuperFastPython.com where you can find hundreds of free tutorials on Python concurrency.
And this is why I wrote this book.
100% Money-Back Guarantee
(no questions asked)
I want you to learn asyncio so well that you can confidently use it on current and future projects.
I designed my book to read just like Iโm sitting beside you, showing you how.
I want you to be happy. I want you to win!
I stand behind all of my materials. I know they get results and Iโm proud of them.
Nevertheless, if you decide that my books are not a good fit for you, Iโll understand.
I offer a 100% money-back guarantee, no questions asked.
To get a refund, contact me with your purchase name and email address.
Frequently Asked Questions
This section covers some frequently asked questions.
If you have any questions. Contact me directly. Any time about anything. I will do my best to help.
What are your prerequisites?
This book is designed for Python developers who want to discover how to use and get the most out of asyncio.
Specifically, this book is for:
- Developers that can write simple Python programs.
- Developers that need better performance from current or future Python programs.
- Developers that want to learn asynchronous programming in Python.
This book does not require that you are an expert in the Python programming language or concurrency.
Specifically:
- You do not need to be an expert Python developer.
- You do not need to be an expert in concurrency.
What version of Python do you need?
All code examples use Python 3.
Python 3.11+ to be exact.
Python 2.7 is not supported because it reached its โend of lifeโ in 2020.
Are there code examples?
Yes.
There are 103 .py code files.
Each lesson has one or more complete, standalone, and fully working code examples.
The book is provided in a .zip file that includes a src/ directory containing all source code files used in the book.
How long will the book take you to finish?
About 1 hour per chapter.
Work at your own pace.
I recommend 1 chapter per day, over about 36 days (about 1 month).
Thereโs no rush and I recommend that you take your time.
The book is designed to be read linearly from start to finish, guiding you from being a Python developer at the start of the book to being a Python developer who can confidently use asyncio in your projects by the end of the book.
To avoid overload, I recommend completing one lesson per day, such as in the evening or during your lunch break. This will allow you to complete the transformation in about one week.
I recommend you maintain a directory with all of the code you type from the lessons in the book. This will allow you to use the directory as your own private code library, allowing you to copy-paste code into your projects in the future.
I recommend trying to adapt and extend the examples in the lessons. Play with them. Break them. This will help you learn more about how the API works and why we follow specific usage patterns.
What format is the book?
You can read the book on your screen, next to your editor.
You can also read the book on your tablet, away from your workstation.
The ebook is provided in 2 formats:
- PDF (.pdf): perfect for reading on the screen or tablet.
- EPUB (.epub): perfect for reading on a tablet with a Kindle or iBooks app.
Many developers like to read technical books on their tablet or iPad.
How can you get more help?
The chapters in this book were designed to be easy to read and follow.
Nevertheless, sometimes we need a little extra help.
A list of further reading resources is provided at the end of each lesson. These can be helpful if you are interested in learning more about the topic covered, such as fine-grained details of the standard library and API functions used.
The conclusions at the end of the book provide a complete list of websites and books that can help if you want to learn more about Python concurrency and the relevant parts of the Python standard library. It also lists places where you can go online and ask questions about Python concurrency.
Finally, if you ever have questions about the lessons or code in this book, you can contact me any time and I will do my best to help. My contact details are provided at the end of the book.
How many pages is the book?
The PDF is 388 US letter-sized pages.
Can you print the book?
Yes.
Although, I think itโs better to work through it on the screen.
- You can search, skip, and jump around really fast.
- You can copy and paste code examples.
- You can compare code output directly.
Is there digital rights management?
No.
The ebooks have no DRM.
Do you get free updates?
Yes.
I update all of my books all of the time to fix typos and to adjust to changes to the APIs with each new release of Python.
You can also email me any time and I will send you the latest version.
Can you buy the book elsewhere?
Yes!
You can get a Kindle or paperback version from Amazon.
Many developers prefer to buy from the Kindle store on Amazon directly.
Below is a photo of Python Asyncio Mastery on my kindle.
In fact, the book was an Amazon #1 Best Seller on the day it was released:
Can you get a paperback version?
Yes!
You can get a paperback version from Amazon.
Can you read a sample?
Yes.
You can read a book sample via Google Books โpreviewโ or via the Amazon โlook insideโ feature:
Generally, if you like my writing style on SuperFastPython, then you will like the books.
Does asyncio work on your operating system?
Yes.
Python concurrency is built into the Python programming language and works equally well on:
- Windows
- macOS
- Linux
Does asyncio work on your hardware?
Yes.
Python concurrency is agnostic to the underlying CPU hardware.
If you are running Python on a modern computer, then you will have support for concurrency, e.g. Intel, AMD, ARM, and Apple Silicon CPUs are supported.
How does the โPython Asyncio Masteryโ book compare to the โPython Asyncio Jump-Startโ book?
I wrote another book on asyncio titled โPython Asyncio Jump-Startโ. It is still current, useful, and popular, and it is very different from this โPython Asyncio Masteryโ book.
Letโs compare them directly:
Python Asyncio Mastery
- 36 chapters
- 103 complete code examples
- 388 pages
Python Asyncio Jump-Start
- 7 lessons
- 27 complete code examples
- 131 pages
The Python Asyncio Mastery book is much longer and covers more of the API. For example, a small section on an API feature in Python Asyncio Jump-Start might be a whole chapter in Python Asyncio Mastery with full code examples.
I recommend:
- Python Asyncio Mastery: If you want to master the high-level asyncio API in the Python standard library.
- Python Asyncio Jump-Start: If you want a taste of asyncio.
What Are You Waiting For?
Stop reading outdated StackOverflow answers.
Learn Python concurrency correctly, step-by-step.
Start today.
Buy now and get your copy in seconds!
Get your copy now:
All prices are in USD.
(also get the book from Amazon, GumRoad, and GooglePlay stores)