Run Parallel and Asynchronous Tasks, Once You Learn The ProcessPoolExecutor
Imagine developing Python programs that effortlessly scaled with the number of CPU cores available in your system
Your python code does not have to be slow! (or run on just one cpu)
If you can invest as little as one hour per day, then in one week you will know how to confidently bring parallel programming to your current and future projects.
Convinced? Jump to the book, otherwise read onโฆ
How Much Faster Could Your Code Run
(if it used all CPU cores)?
Is this situation familiar to youโฆ
You have a slow for-loop in your program that executes tasks one-by-one.
It waits for each task to complete before executing the next.
Yet, you have 2, 4, 8 or more CPU cores sitting idle.
Using electricity.
Waiting for work.
What a waste!
What if you could use all of the CPU cores in your system right now, with just a very small change to your code.
- You could change a slow sequential for-loop into a blazingly-fast parallel for-loop.
- You could change run-and-wait tasks into fire-and-forget asynchronous tasks.
Imagine dropping run times down by 25%, 50% or more.
This is possible right now with a lesser-known Python class that offers super-easy-to-use process-based concurrency (and is already installed on your system).
The Path to Faster Python Code
(is concurrency)
Python is a joy to use, but getting Python code to run fast is challenging.
Concurrency is a standard approach to running multiple functions simultaneously.
Python concurrency has a bad reputation. So bad, that many believe Python does not support true concurrency.
Iโm happy to say that these misconceptions are dead wrong.
Python supports real concurrency with first-class native support for thread-based and process-based concurrency.
It always has.
On all major platforms, like Windows, macOS, and Linux.
And most importantly, Python concurrency is easy and fun to use.
The trick is to use process pools.
ProcessPoolExecutor
The Modern Class For Parallel Python
The ProcessPoolExecutor class provides easy-to-use process-based concurrency.
This is not some random third-party library, this is a class provided in the Python standard library (already installed on your system).
The ProcessPoolExecutor class has been in the standard library since Python 3.2.
This is the class you need to use to make your code run faster.
It is specifically designed for you to run for-loops in parallel and automatically use all of the CPU cores available in your system.
Thereโs just one problem.
No one knows about it (or how to use it well).
- The API documentation is thin at best, providing very little guidance on how to use its features.
- The API docs are buried deep within the Python documentation, making it impossible to find.
- The few examples out there on the web are terse and years out of date.
This is madness!
The perfect class for faster parallel Python code is right there in the standard library, and very few people know it exists or can locate it, let alone decipher the API documentation.
Introducing:
โProcessPoolExecutor Jump-Startโ
โPython ProcessPoolExecutor Jump-Startโ is my new book that will teach you how to develop parallel loops in Python, from scratch.
This book distills only what you need to know to get started and be effective with the ProcessPoolExecutor class, super fast.
Itโs exactly how I would teach you to use ProcessPoolExecutor if we were sitting together, pair programming.
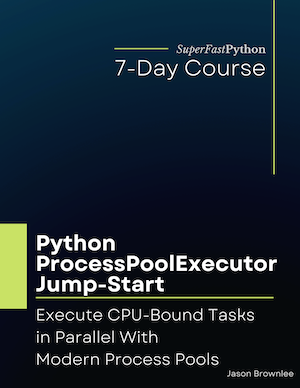
Technical Details:
- 7 lessonsย taught by example with full working code listings.
- 31 .py code filesย included that you can use as templates
- 96 pagesย for on-screen reading open next to your editor.
- 2 formats (PDF and EPUB)ย for screen, tablet, and kindle reading.
- 2 megabyteย .zip download that contains the ebook and all source code.
โPython ProcessPoolExecutor Jump-Startโ will lead you on the path from a Python developer interested in concurrency to a developer that can confidently develop programs using the ProcessPoolExecutor class.
- No fluff.
- Just concepts and code.
- With full working examples.
The book is divided into 7 lessons.
The idea is you can read and work through one lesson per day, and become capable in one week.
It is the shortest and most effective path that I know of transforming you into a Parallel Python Engineer.
Choose your package:
BOOK
You get the book:
- ProcessPoolExecutor Jump-Start
PDF and EPUB formats
Includes all source code files
BOXED SET
TOP SELLER
You get all 7 books:
- Threading Jump-Start
- Multiprocessing Jump-Start
- Asyncio Jump-Start
- ThreadPool Jump-Start
- Pool Jump-Start
- ThreadPoolExecutor Jump-Start
- ProcessPoolExecutor Jump-Start
(that is a 9.52% discount)
BOOKSHELF
BEST VALUE
You get everything (15+ books):
- Threading Jump-Start
- Multiprocessing Jump-Start
- Asyncio Jump-Start
- ThreadPool Jump-Start
- Pool Jump-Start
- ThreadPoolExecutor Jump-Start
- ProcessPoolExecutor Jump-Start
- Threading Interview Questions
- Multiprocessing Interview Questions
- Asyncio Interview Questions
- Executors Interview Questions
- Concurrent File I/O
- Concurrent NumPy
- Python Benchmarking
- Python Asyncio Mastery
Bonus, you also get:
- Concurrent For Loops Guide
- API Mind Maps (4)
- API Cheat Sheets (7)
That is $210 of Value!
(you get a 10.95% discount)
All prices are in USD.
(also get the book from Amazon, GumRoad, and GooglePlay stores)
See What Customers Are Saying:
Lars Klemstein
A really good introduction to Python parallel processing on the right technical level.
Richard Palladino
The SuperFastPython series was exactly what I needed. The information was concise. I was able to understand things much quicker than any other lesson, tutorial, or resource Iโd used before. I worked through a lot of the problems I had in the past with my particular use-case and also discovered some things just werenโt possible unless I wanted to recode the dependencies.
I was able to test it and double my speed compared to the BEST results of my past concurrency and async approaches.
Anthony Wilson
Clear. Insightful. Helped me develop from scratch a fully async system used in production
Greatly eases the learning curve for multitasking in Python
ASYNCIO is one Python subsystem for simple multitasking. I wouldnโt classify this library as fully mature and I was struggling greatly to absorb the concepts behind the function calls. I also found it difficult to get the function call syntax right without a bunch of trial-an-error.
I realized that at some point I would need to move some of my tasks to different multi-tasking subsystems. Iโd been using the authorโs web site for help and discovered the book set. It has provided me with both perspective and practical application of ASYNCIO.
If you donโt have a lot of time to waste learning about this topic and you arenโt into pain, Iโd recommend the book set. Well worth the money for me.
Really good!
The whole series of books from Jason is really good. All concepts are well explained, he goes in-depth with clear examples.
Robert Young
Miลกel Sabo
I succeed to faster my code using of Process pool 2.3 times. After some additional optimization I have succeed to speed up to 10 times.
Tomex Iskandar
You Get 7 Laser-Focused Lessons
This book is divided into 7 lessons, they are:
- Lesson 01: Processes, Executors, and Process Pools
- What is Process-Based Concurrency
- Process Pools Provide Reusable Workers
- ProcessPoolExecutor for Python Process Pools
- Welcome to the ProcessPoolExecutor Class
- When to Use the ProcessPoolExecutor
- Lesson 02: Configure the ProcessPoolExecutor
- How to Configure the ProcessPoolExecutor
- How to Inspect the Default Configuration
- How to Configure the Number of Worker Processes
- How to Configure the Multiprocessing Context
- How to Configure Worker Process Initialization
- Lesson 03: Execute Multiple Tasks Concurrently
- What is the map() Method
- How to Execute Tasks with One Argument
- How to Execute Tasks with Multiple Arguments
- How to Execute Tasks with No Return Values
- How to Execute Tasks with a Timeout
- How to Execute Tasks in Chunks
- Lesson 04: Execute One-Off Tasks Asynchronously
- What is the submit() Method
- How to Execute a Task with One Argument
- How to Execute a Task with Multiple Arguments
- How to Execute a Task with No Arguments
- How to Execute a Task with No Return Value
- How to Execute Many One-Off Tasks
- Lesson 05: Query Asynchronous Tasks
- What are Future Objects
- How to Check the Status of Future Objects
- How to Get Results From Future Objects
- How to Cancel Future Objects
- How to Add Callbacks to Future Objects
- How to Get Exceptions From Future Objects
- Lesson 06: Manage Collections of Asynchronous Tasks
- What are the Module Functions
- How to Handle Results as Tasks Finish
- How to Wait for All Tasks
- How to Wait for the First Task
- How to Wait for First Task Failure
- Lesson 07: Case Study Calculate Fibonacci Numbers
- How to Calculate Fibonacci Numbers Sequentially (slow)
- How to Calculate Fibonacci Numbers in Parallel (fast)
Next, let's look at the structure of each lesson.
Highly-structured lessons on how you can get results
Each lesson has two main parts, they are:
- 1) The lesson body that teaches the concepts and working code examples.
- 2) The lesson review that summarizes what was learned with exercises.
The body of the lesson will introduce a topic with code examples, whereas the lesson overview will review what was learned with exercises and links for further information.
Each lesson has a specific learning outcome and is designed to be completed in about one hour.
Each lesson is also designed to be self-contained so that you can read the lessons out of order if you choose, such as dipping into topics in the future to solve specific programming problems.
We Python developers learn best from real and working code examples. So each lesson has multiple large worked examples with sample output.
Next, let's look at what you will know after finishing the book.
Your Learning Outcomes
Transform from "python developer" into "Python developer that can create scalable and parallel programs using the ProcessPoolExecutor"
After working through all of the lessons in this book, you will know:
- How to create a ProcessPoolExecutor to execute CPU-bound tasks concurrently.
- How to configure the ProcessPoolExecutor including inspecting the default configuration, how to set the number of worker processes, process start method, and how to initialize worker processes.
- How to execute multiple tasks concurrently using the map() method.
- How to issue asynchronous tasks to the ProcessPoolExecutor using the submit() method.
- How to query, get results, handle exceptions and use callback functions with Future objects for asynchronous tasks.
- How to manage collections of asynchronous tasks issued to the ProcessPoolExecutor including how to handle results in task completion order and wait for all tasks or for the first task to complete.
- How to build upon what you have learned to speed up the CPU-bound task of calculating Fibonacci numbers by calculating them in parallel and then further optimizing the parallel execution of the program.
Get your copy now:
100% Money-Back Guarantee
(no questions asked)
I want you to actually learn the Python ProcessPoolExecutor so well that you can confidently use it on current and future projects.
I designed my book to read just like I'm sitting beside you, showing you how.
I want you to be happy. I want you to win!
I stand behind all of my materials. I know they get results and I'm proud of them.
Nevertheless, if you decide that my books are not a good fit for you, I'll understand.
I offer a 100% money-back guarantee, no questions asked.
To get a refund, contact me with your purchase name and email address.
Frequently Asked Questions
This section covers some frequently asked questions.
If you have any questions. Contact me directly. Any time about anything. I will do my best to help.
What are your prerequisites?
This book is designed for Python developers who want to discover how to use and get the most out of the Python ProcessPoolExecutor.
Specifically, this book is for:
- Developers that can write simple Python programs.
- Developers that need better performance from current or future Python programs.
- Developers that want to learn concurrent programming in Python.
This book does not require that you are an expert in the Python programming language or concurrency.
Specifically:
- You do not need to be an expert Python developer.
- You do not need to be an expert in concurrency.
What version of python do you need?
All code examples use Python 3.
Python 3.9+ to be exact.
Python 2.7 is not supported because it reached its "end of life" in 2020.
Are there code examples?
Yes.
There are 31 .py code files.
Each lesson has one or more complete, standalone, and fully-working code examples.
The book is provided in a .zip file that includes a src/ directory containing all source code files used in the book.
How long will the book take you to finish?
About 1 hour per lesson.
Work at your own pace.
I recommend 1 lesson per day, over 7 days (1 week).
There's no rush and I recommend that you take your time.
The book is designed to be read linearly from start to finish, guiding you from being a Python developer at the start of the book to being a Python developer that can confidently use the Python ProcessPoolExecutor in your projects by the end of the book.
In order to avoid overload, I recommend completing one lesson per day, such as in the evening or during your lunch break. This will allow you to complete the transformation in about one week.
I recommend you maintain a directory with all of the code you type from the lessons in the book. This will allow you to use the directory as your own private code library, allowing you to copy-paste code into your projects in the future.
I recommend trying to adapt and extend the examples in the lessons. Play with them. Break them. This will help you learn more about how the API works and why we follow specific usage patterns.
What format is the book?
You can read the book on your screen, next to your editor.
You can also read the book on your tablet, away from your workstation.
The ebook is provided in 2 formats:
- PDF (.pdf): perfect for reading on the screen or tablet.
- EPUB (.epub): perfect for reading on a tablet with a Kindle or iBooks app.
Many developers like to read technical books on their tablet or iPad.
Below is a photo of the epub version of the book on my iPad.
How can you get more help?
The lessons in this book were designed to be easy to read and follow.
Nevertheless, sometimes we need a little extra help.
A list of further reading resources is provided at the end of each lesson. These can be helpful if you are interested in learning more about the topic covered, such as fine-grained details of the standard library and API functions used.
The conclusions at the end of the book provide a complete list of websites and books that can help if you want to learn more about Python concurrency and the relevant parts of the Python standard library. It also lists places where you can go online and ask questions about Python concurrency.
Finally, if you ever have questions about the lessons or code in this book, you can contact me any time and I will do my best to help. My contact details are provided at the end of the book.
How many pages is the book?
The PDF is 98 pages.
Can you print the book?
Yes.
Although, I think it's better to work through it on the screen.
- You can search, skip, and jump around really fast.
- You can copy and paste code examples.
- You can compare code output directly.
Is there digital rights management?
No.
The ebooks have no DRM.
Do you get free updates?
Yes.
Each time I release an updated version, I will send you an email with a link so that you can download the latest version for free.
You can also email me any time and I will send you the latest version.
Can you buy the book elsewhere?
Yes!
You can get a kindle or paperback version from Amazon.
Many developers prefer to buy from the kindle store on amazon directly.
Can you get a paperback version of the book?
Yes!
You can get a paperback version from Amazon.
Can you read a sample of the book?
Yes.
You can read a book sample via google books "preview" or via the amazon "look inside" feature:
Generally, if you like my writing style on SuperFastPython, then you will like the books.
Can you download the source code now?
The source code (.py) files are included in the .zip with the book.
Nevertheless, you can also download all of the code from the dedicated GitHub Project:
Does the ProcessPoolExecutor work on your operating system?
Yes.
Python concurrency is built into the Python programming language and works equally well on:
- Windows
- macOS
- Linux
Does the ProcessPoolExecutor work on your hardware?
Yes.
Python concurrency is agnostic to the underlying CPU hardware.
If you are running Python on a modern computer, then you will have support for concurrency, e.g. Intel, AMD, ARM, and Apple Silicon CPUs are supported.
How does the "Jump-Start Book" compare to the "Complete Guides"?
The SuperFastPython Jump-Start books are laser-focused on making you productive with a Python concurrency module or class as fast as possible.
This means that many broader topics are not covered because they are not on the critical path.
The guides on SuperFastPython.com are broader in scope and compare the class or module to related modules, describing best practices, common errors, common usage questions, and common objects.
This material may be interesting but is a distraction when you are focused on getting productive as fast as possible.
Another important difference is that the jump-start books are provided in book form, whereas the guides are very long web pages.
This makes the books easy to read on a kindle, tablet, or paperback, as well as the screen, whereas the guides must be read in the browser.
About the Author
Hi, I'm Jason Brownlee, Ph.D.
I'm a Python developer, husband, and father to two boys.
I want to share something with you.
I am obsessed with Python concurrency, but I wasn't always this way.
My background is in Artificial Intelligence and I have a few fancy degrees and past job titles to prove it.
You can see my LinkedIn profile here:
- Jason Brownlee LinkedIn Profile
(follow me if you like)
So what?
Well, AI and machine learning has been hot for the last decade. I have spent that time as a Python machine learning developer:
- Working on a range of predictive modeling projects.
- Writing more than 1,000+ tutorials.
- Authoring over 20+ books.
There's one thing about machine learning in Python, your code must be fast.
Really fast.
Modeling code is already generally fast, built on top of C and Fortran code libraries.
But you know how it is on real projectsโฆ
You always have to glue bits together, wrap the fast code and run it many times, and so on.
Making code run fast requires Python concurrency and I have spent most of the last decade using all the different types of Python concurrency available.
Including threading, multiprocessing, asyncio, and the suite of popular libraries.
I know my way around Python concurrency and I am deeply frustrated at the bad wrap it has.
This is why I started SuperFastPython.com where you can find hundreds of free tutorials on Python concurrency.
And this is why I wrote this book.
Praise for Super Fast Python
Python developers write to me all the time and let me know how helpful my tutorials and books have been.
Below are some select examples posted to LinkedIn.
What Are You Waiting For?
Stop reading outdated StackOverflow answers.
Learn Python concurrency correctly, step-by-step.
Start today.
Buy now and get your copy in seconds!